Junit Class Annotation Java – In this tutorial we will see how to write Junit for a class where Annotation has been used. This is needed if you are using annotation in your class. Now a days annotation is key for any project and multiple class uses annotation. If you are working on project where some annotation is mandatory to have on the class then you can validate using JUNIT scanning complete package to find if all class in the package has Annotation available. In my project this was one the mandatory thing to do where every model class was having JSON annotation because we were creating Java to JSON Vice versa. Please have steps below:
1. To test we will have to create small project. You can use any existing project as well. I believe you came here because you were looking to create JUNIT of the class which is has annotation in already existing project. If not then you can create similar Maven project as below. This is just for reference if you want all code of this project then in the end I have given Git repository link:
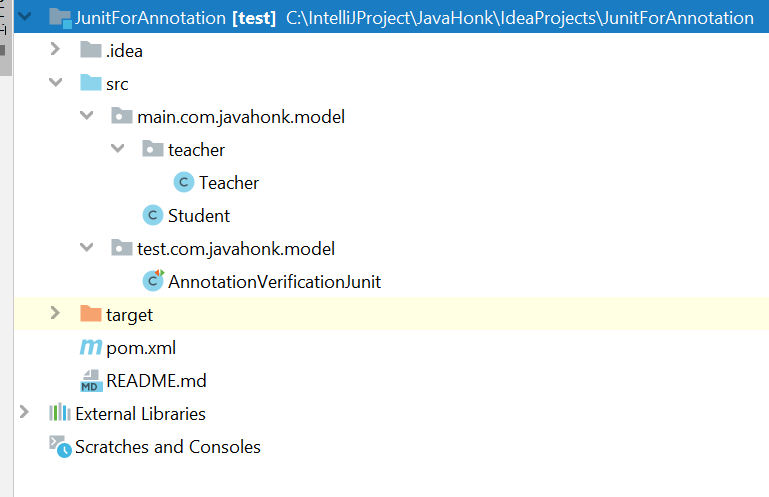
2. As you see above we have created Maven project with two model class: Teacher and Student. Below class AnnotationVerificationJunit.java is a test class which will validate if both class Teacher and Student is having annotation or not and not only it will check annotation but it will also validate the class name of the annotation:
AnnotationVerificationJunit.java
package test.com.javahonk.model;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
import org.junit.Assert;
import org.junit.Before;
import org.junit.Test;
import org.reflections.Reflections;
import org.reflections.scanners.SubTypesScanner;
import java.lang.annotation.Annotation;
import java.util.Iterator;
import java.util.Set;
public class AnnotationVerificationJunit {
private Set<Class<? extends Object>> allClasses;
@Before
public void init() {
allClasses = getClassesFromPakcage("main.com.javahonk.model");
}
@Test
public void testAnnotation() throws Exception {
Iterator it = allClasses.iterator();
Class clazz = null;
while (it.hasNext()) {
clazz = (Class) it.next();
Annotation jsonInclue = clazz.getDeclaredAnnotation(JsonInclude.class);
Annotation jsonIgnoreProperties = clazz.getDeclaredAnnotation(JsonIgnoreProperties.class);
Annotation jsonAutoDetectAnnotation = clazz.getAnnotation(JsonAutoDetect.class);
Assert.assertNotNull("jsonInclueAnnotation not found: " + clazz.getName(), jsonInclue);
Assert.assertNotNull("jsonIgnoreProperties not found: " + clazz.getName(), jsonIgnoreProperties);
Assert.assertNotNull("jsonAutoDetectAnnotation not found: " + clazz.getName(), jsonAutoDetectAnnotation);
}
}
public static Set<Class<?>> getClassesFromPakcage(String path){
Reflections reflections = new Reflections(path, new SubTypesScanner(false));
Set<Class<? extends Object>> allClasses = reflections.getSubTypesOf(Object.class);
return allClasses;
}
}
3. This is Teacher.java with annotation:
package main.com.javahonk.model.teacher;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@JsonIgnoreProperties(ignoreUnknown =true)
@JsonAutoDetect(
fieldVisibility = JsonAutoDetect.Visibility.ANY, getterVisibility = JsonAutoDetect.Visibility.NONE,
setterVisibility = JsonAutoDetect.Visibility.NONE, creatorVisibility = JsonAutoDetect.Visibility.NONE
)
public class Teacher {
private String firstName;
private String lastName;
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
@Override
public String toString() {
return "Student{" +
"firstName='" + firstName + '\'' +
", lastName='" + lastName + '\'' +
'}';
}
}
4. Student.java also has annotation which we will check through JUNIT:
package main.com.javahonk.model;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
import java.io.Serializable;
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@JsonIgnoreProperties(ignoreUnknown =true)
@JsonAutoDetect(
fieldVisibility = JsonAutoDetect.Visibility.ANY, getterVisibility = JsonAutoDetect.Visibility.NONE,
setterVisibility = JsonAutoDetect.Visibility.NONE, creatorVisibility = JsonAutoDetect.Visibility.NONE
)
public class Student implements Serializable {
private String firstName;
private String lastName;
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
@Override
public String toString() {
return "Student{" +
"firstName='" + firstName + '\'' +
", lastName='" + lastName + '\'' +
'}';
}
}
5. I kept this file which is Maven project pom.xml with all the dependencies if you need it and try to understand what are dependencies are needed. If you are using sample project from above then you need this file:
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-annotations</artifactId>
<version>2.9.8</version>
</dependency>
<dependency>
<groupId>org.reflections</groupId>
<artifactId>reflections</artifactId>
<version>0.9.11</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>compile</scope>
</dependency>
</dependencies>
6. Junit Class Annotation Java – To Download complete project link I have give GIT repo link below:
https://github.com/javahonk/JunitForAnnotation