Java Map Keep Insertion Order – If you are looking to do implementation of Map using java where ordering of the object needs to be preserved then map is not a right option as this class does not makes any guarantees of the order of the object.
I will suggest to use LinkedHashMap or TreeMap. Below is reason:
Behavior | TreeMap | LinkedHashMap | HashMap |
Implementation | Red-Black Tree | double-linked buckets | buckets |
Get/put remove containsKey | O(log(n)) | O(1) | O(1) |
Iteration order | sorted according to the natural ordering | insertion-order | no guarantee of order |
Interfaces | NavigableMap SortedMap Map | Map | Map |
Null | only values | allowed | allowed |
synchronized | Not synchronized | Not synchronized | Not synchronized |
Fail-fast | Not guaranteed due to unsynchronized concurrent modification | Not guaranteed due to unsynchronized concurrent modification | Not guaranteed due to unsynchronized concurrent modification |
LinkedHashMap keeps keys in the order the way it was inserted and on the other hand TreeMap also provide same feature but internally it uses comparator to sort to maintain ordering of the object. Lets see by example below:
LinkedHashMapExample.java
import java.util.LinkedHashMap;
import java.util.Set;
public class LinkedHashMapExample {
public static void main(String[] args) {
LinkedHashMap<Integer, String> map = new LinkedHashMap<>();
map.put(1, "Javahonk1");
map.put(2, "Javahonk2");
map.put(3, "Javahonk3");
map.put(4, "Javahonk4");
Set<Integer> keys = map.keySet();
Integer[] arrayValue = keys.toArray(new Integer[0]);
for (int i = 0; i < arrayValue.length; i++) {
if (new Integer(i + 1).equals(arrayValue[i])) {
System.out.println("For loop count: " + new Integer(i + 1)
+ " LinkedHashMap Key: " + arrayValue[i]);
}
}
}
}
Output:
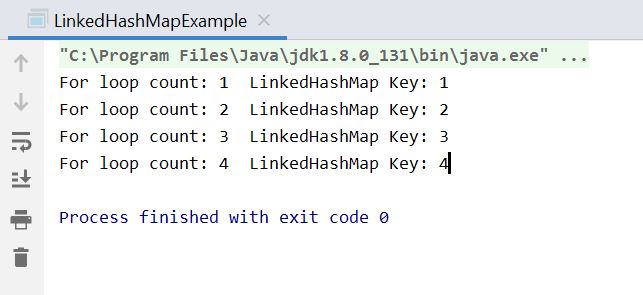
TreeMapExample.java
import java.util.Set;
import java.util.TreeMap;
public class TreeMapExample {
public static void main(String args[]){
TreeMap<Integer, String> map = new TreeMap<Integer, String>();
map.put(1, "Javahonk1");
map.put(2, "Javahonk2");
map.put(3, "Javahonk3");
map.put(4, "Javahonk4");
map.put(5, "Javahonk5");
Set<Integer> keys = map.keySet();
Integer[] arrayValue = keys.toArray(new Integer[0]);
for (int i = 0; i < arrayValue.length; i++) {
if (new Integer(i + 1).equals(arrayValue[i])) {
System.out.println("For loop count: " + new Integer(i + 1)
+ " TreeMap Key: " + arrayValue[i]);
}
}
}
}
Output:
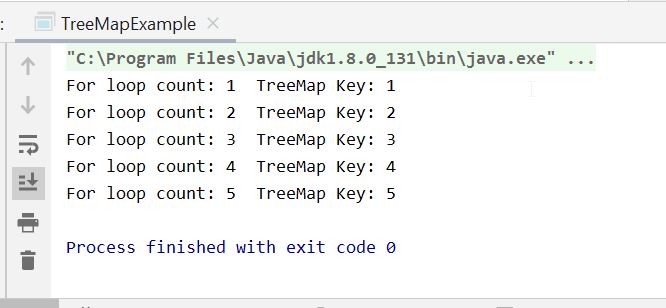
Java Map Keep Insertion Order Reference: Oracle Java LinkedHashMap and TreeMap