Spring Boot HTTPS Application – Spring Boot is very powerful and easy to create stand-alone, production ready Spring application can be just configured and run. Some of its feature is:
1. Quickly you can created stand-alone application.
2. Its embedded with Jetty, Tomcat which can be just run no need to create WAR file and deploy on tomcat or any application server
3. Its automatically add third party library if needed
4. No code generation and there is no need for XML configuration
5. Its gives you production ready code with metric and very importantly health check and you can externalize all configuration which is very demanding for any production application
6. Its simply build configuration and opinionated starter dependencies to simplify application
7. Here we will create simple Spring boot application HTTPS enabled.
What you need:
Java 8, Maven any latest version , Spring Boot latest release and Self-Signed certificate (PKCS12)
Project structure:
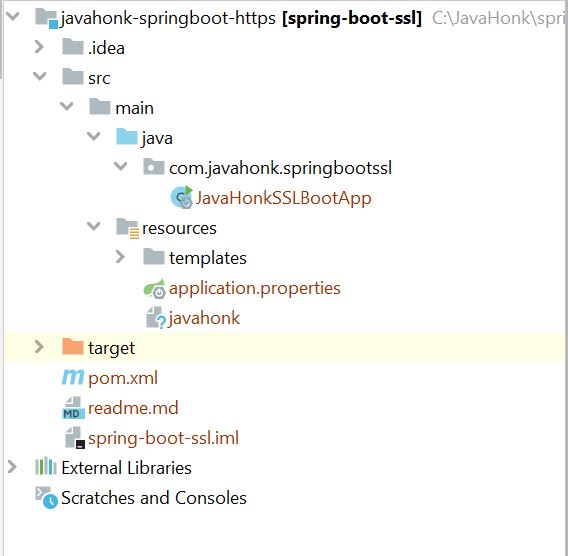
To run this application you will have to generate Self-signed Certificate and to do this you can use keytool which generate certificaiton in PKCS12 format. Below is command:
C:\Program Files\Java\jdk1.8.0_131\bin>keytool -genkeypair -keyalg RSA -keysize 2048 -storetype PKCS12 -keystore javahonk -validity 400
Enter keystore password:
Re-enter new password:
What is your first and last name?
[Unknown]: javahonk
What is the name of your organizational unit?
[Unknown]: javahonk
What is the name of your organization?
[Unknown]: javahonk
What is the name of your City or Locality?
[Unknown]: javahonk
What is the name of your State or Province?
[Unknown]: javahonk
What is the two-letter country code for this unit?
[Unknown]:
Is CN=javahonk, OU=javahonk, O=javahonk, L=javahonk, ST=javahonk, C=Unknown correct?
[no]: yes
This will generate certification in same root directory copy to resource folder
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.javhonk.springbootssl</groupId>
<artifactId>springbootssl</artifactId>
<version>1.0</version>
<name>javahonk-springboot-https</name>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.2.4.RELEASE</version>
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<optional>true</optional>
</dependency>
</dependencies>
<build>
<finalName>spring-boot-web</finalName>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
<configuration>
<source>${java.version}</source>
<target>${java.version}</target>
</configuration>
</plugin>
</plugins>
</build>
</project>
Starter application
package com.javahonk.springbootssl;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
@SpringBootApplication
public class JavaHonkSSLBootApp {
public static void main(String[] args) {
SpringApplication.run(JavaHonkSSLBootApp.class, args);
}
@GetMapping("/")
public String index(final Model model) {
model.addAttribute("javahonk_title", "First Spring Boot with HTTPS and (SSL)");
model.addAttribute("javahonk_msg", "Wow! Page is loaded successfully");
model.addAttribute("javahonk_dottedLine", "---------------------------------------");
return "javahonk";
}
}
application.properties file
# SSL
# Spring Security
# security.require-ssl=true
# PKCS12 or JKS
server.ssl.keyStoreType=PKCS12
server.port=8443
server.ssl.key-store=classpath:javahonk
server.ssl.key-store-password=javahonk
html file (Note: We are using thymeleaf which is modern server side java template engine for both standalone and web envrionment. its Java/HTML5/XML/XHTML template engine which cna work on both non-web and web environment. Its very well suited for MVC web application. It functionality full Spring framework supported. More you can find here
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<title>Spring Boot with SSL</title>
</head>
<body>
<main role="main" class="container">
<div class="starter-template">
<h1 th:text="${javahonk_dottedLine}">javahonk_dottedLine</h1>
<h1 th:text="${javahonk_title}">Title</h1>
<p th:text="${javahonk_msg}">Message</p>
<h1 th:text="${javahonk_dottedLine}">javahonk_dottedLine</h1>
</div>
</main>
</body>
</html>
To run this application right click JavaHonkSSLBootApp.java:
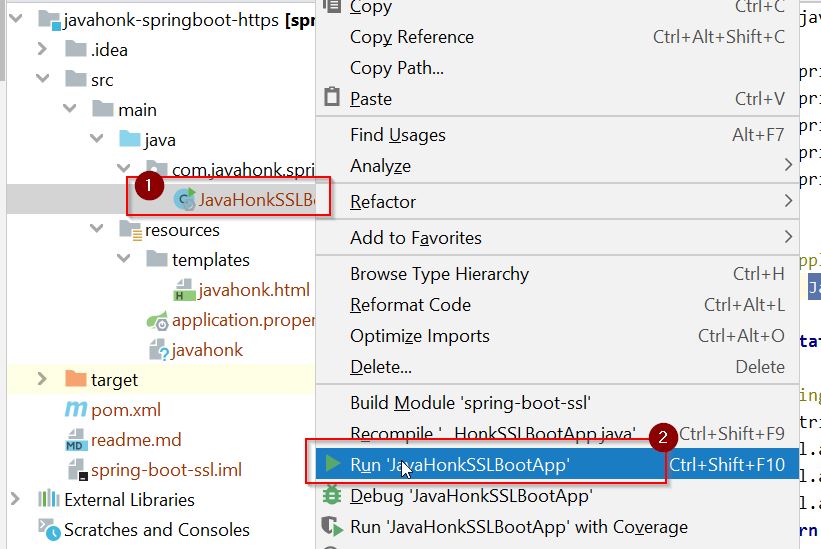
Once application is started go to browser and type: https://localhost:8443
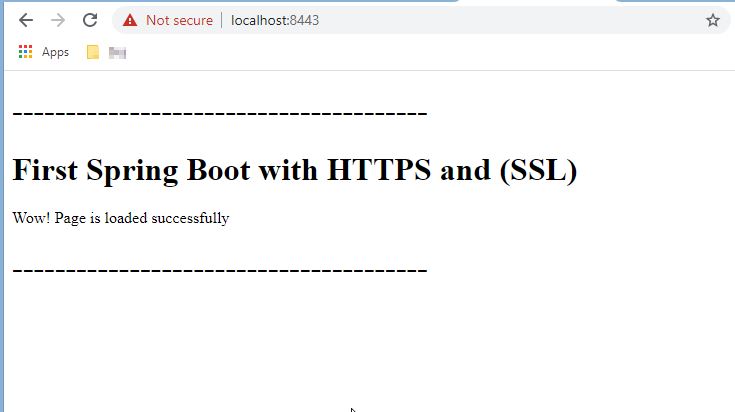