Geometry point in rectangle: Write a program that prompts the user to enter a point (x, y) and checks whether the point is within the rectangle centered at (0, 0) with width 10 and height 5. For example below:
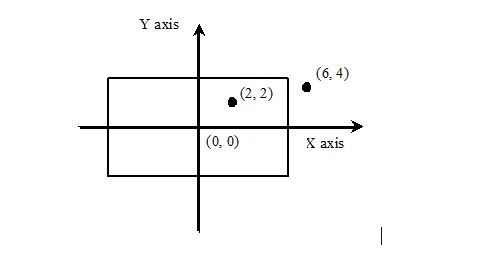
Sample 1:
Enter a point with two coordinates: 3 1
Point (3.0, 1.0) is in the rectangle
Sample 2:
Enter a point with two coordinates: 7 7
Point (7.0, 7.0) is not in the rectangle
import java.util.Scanner;
public class Exercise_03_23 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
// Prompt the user to enter a point (x, y)
System.out.print("Enter a point with two coordinates: ");
double x = input.nextDouble();
double y = input.nextDouble();
// Check whether the point is within the rectangle
// centered at (0, 0) with width 10 and height 5
boolean withinRectangle = (Math.pow(Math.pow(x, 2), 0.5) <= 10 / 2 ) ||
(Math.pow(Math.pow(y, 2), 0.5) <= 5.0 / 2);
// Display results
System.out.println("Point (" + x + ", " + y + ") is " +
((withinRectangle) ? "in " : "not in ") + "the rectangle");
}
}
For more information please visit here